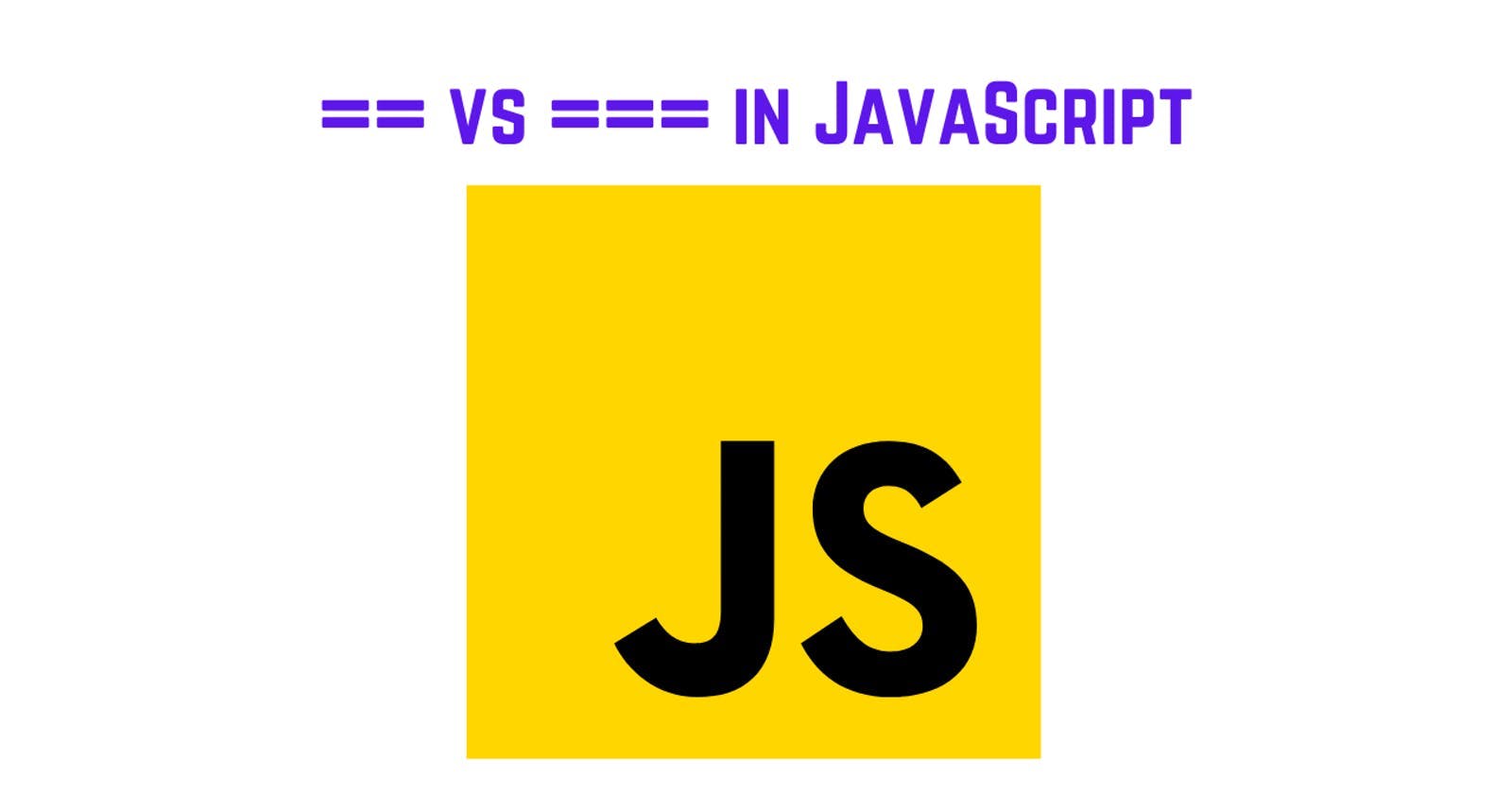
Many times people coming from a different programming language background get confused between == and === operators that we use in JavaScript. This also happens with != and !== operators also. So by the end of this post, you will be able to explain the difference between these operators. So let's get started.
Let's first see, what are comparison operators?
A comparison operator compares its operands and returns a logical value based on whether the comparison is true.
Wait, but the definition doesn't stop here. It comes with some predefined conditions such as:
-
The operands can be numerical, string, objects or logical values.
-
Strings are compared based on standard lexicographical ordering, using Unicode values.
-
If the two operands are different for example, one is numerical and another one is a string then JavaScript attempts to convert them to an appropriate type for the comparison.
-
And this behaviour generally results in comparing the operands numerically.
And now the important one,
- The sole exceptions to type conversion within comparison involve the
===
and!==
operators.
So far we know that ===
and !==
are the exceptions in comparison operators. Now, let's understand why they are exceptions and what is the difference between ==
and ===
of between !=
and !==
.
Understanding ===
Let's consider a variable,
const age = 18;
if(age === 18) console.log(`You are an adult. 🧑 `);
Now, how does this equality operator here in if condition actually works?
Well, just like any other comparison operator it is also a comparison operator so, it will return a true or a false value, so a boolean value, as a result, in case both sides are the same. Let's actually show that to you. If I write in console,
and that's what we use in if condition.
Understanding == .
Now, besides ===
we also have ==
as an equality operator. And this also works as a comparison operator. Have a look.
Difference between == and === .
So the difference is that this one here ===
with triple equal is called the strict equality operator. It is strict because it does not perform type conversion.
So, it returns the true value when both the values are the same.
See the example here,
On the other hand, there is a loose equality operator with only two ==
signs. And the loose equality operator actually does the type conversion. So let's see that again in console,
In this case, we can do "18"
the string equal to the 18
the number and it will still give us true
.
So, again the ==
does not perform type conversion. So what that means is that this "18"
the string here will be converted to the number and then the number 18
is just the same as that of the number 18
on the left-hand side.
Difference between != and !== .
So, as we have talked about the equal-to operators, let's also talk about the inequality operators.
Here we have again two inequality operators one is !=
and the other one is !==
. So, !=
is a loose inequality operator which supports type conversion and !==
is a strict inequality operator which does support type conversion. Let's see it again in the console.
I hope that's not too confusing to you right now. So for comparing values always use the ===
or !==
operator to avoid bugs in your code.
Wrapping Up!
-
The sole exceptions to type conversion within comparison involve the
===
and!==
operators. -
===
and!==
are strict comparisons operators which do not support type conversion. -
==
and!=
are loose comparisons operators which support type conversion. -
Using strict comparison operators in code always helps in avoiding errors.
Thank you!